Create LazyCollection from API resource
Laravel 6 introduced the LazyCollection, a different kind of collection which exploits php generators to save memory usage. While data fetching from database is natively managed by eloquent, Web API-based data fetching is not provided. This article describes how to use a paginated api endpoint to fetch data to a LazyCollection instance.
Tags:
Laravel
api
PHP
generators
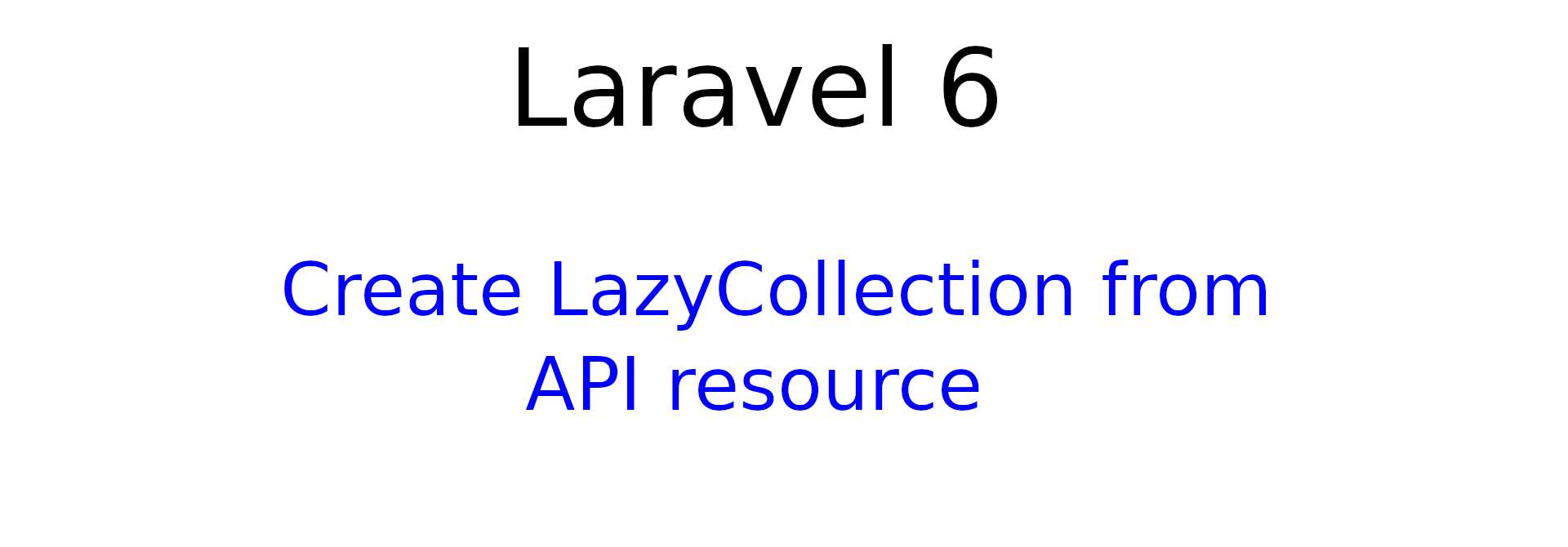
Related posts
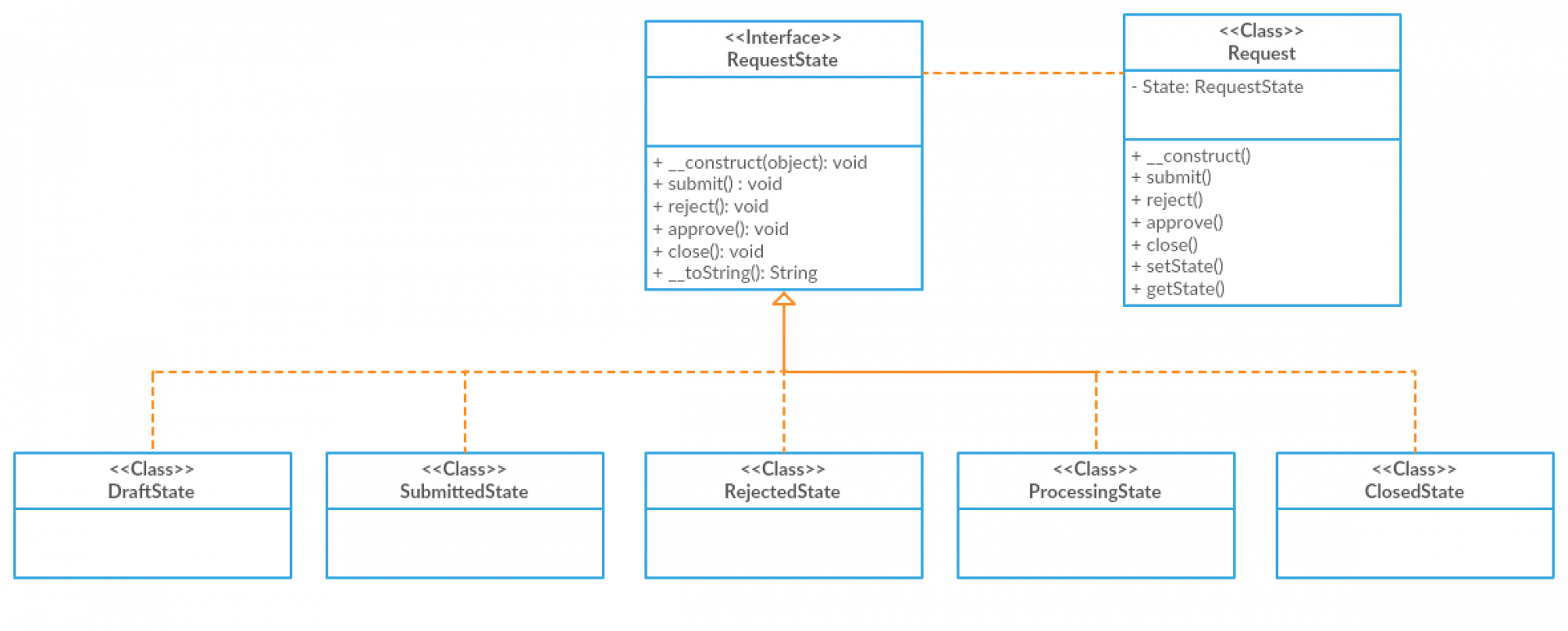
In the previous article TDD IMPLEMENTATION OF FINITE STATE MACHINE (FSM) WITH LARAVEL I discussed a basic approach for implement a Finite State Machine (FSM) on a Laravel model. In this article I will further discuss the topic by applying a more engineered approach. It involves the usage of the State pattern