TDD implementation of Finite State Machine (FSM) with Laravel
This article describes a TDD (Test Driven Development) approach to implement a Finite State Machine (FSM) using PHP+Laravel. However, such method is general and could be used in the programming language of your choice.
Category:
Development
04/07/2018
19863 times
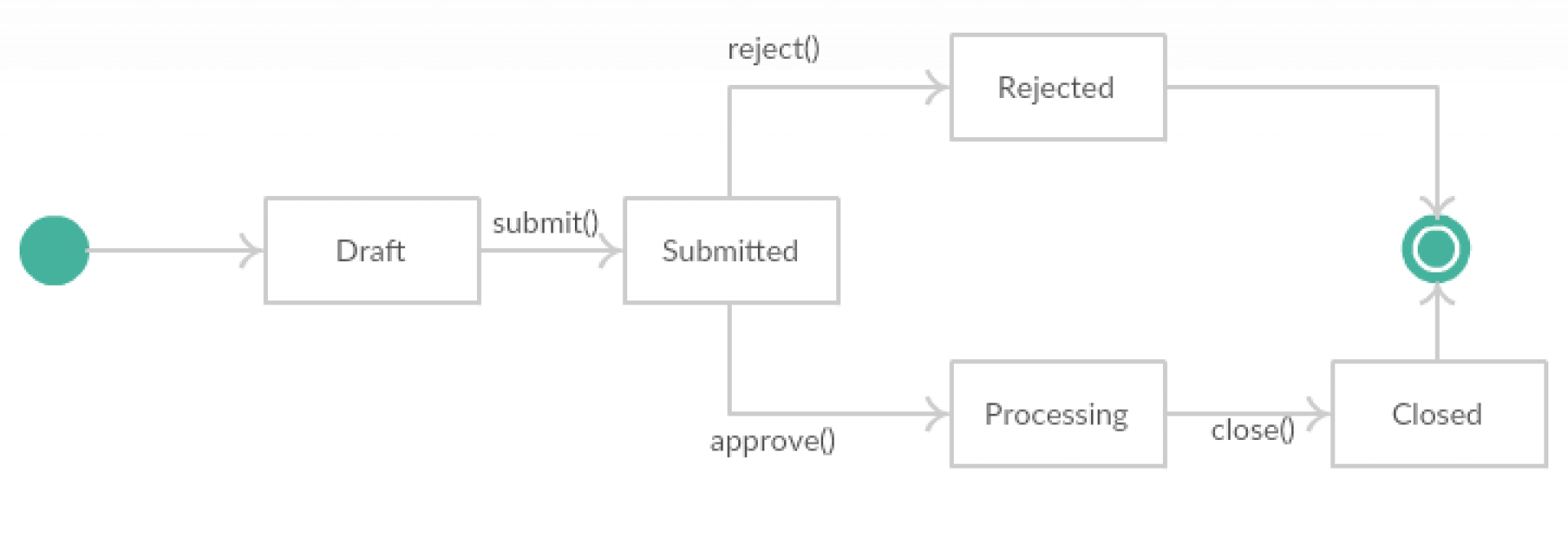
Related posts
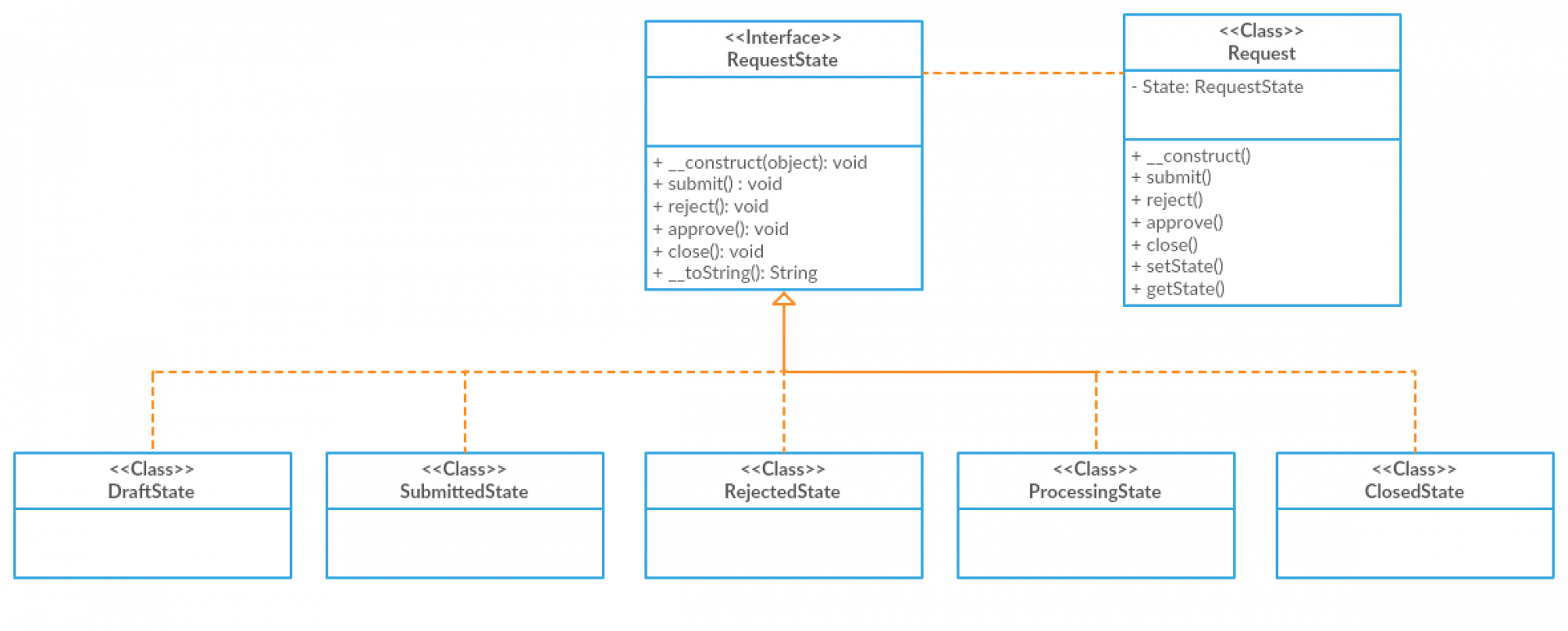
In the previous article TDD IMPLEMENTATION OF FINITE STATE MACHINE (FSM) WITH LARAVEL I discussed a basic approach for implement a Finite State Machine (FSM) on a Laravel model. In this article I will further discuss the topic by applying a more engineered approach. It involves the usage of the State pattern